2025 MySQL Performance Optimization
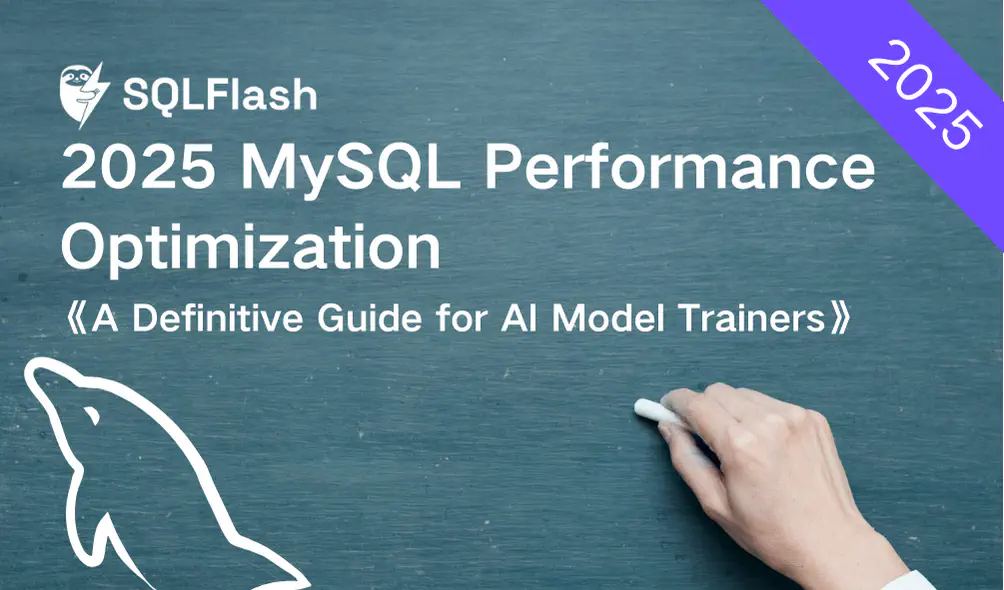
Welcome! This guide helps you make your MySQL database run faster, especially when you’re training AI models. Let’s define some important words:
SQL Tuning: This is like giving your database a tune-up to make it run smoothly. We look at how SQL queries (questions you ask the database) are running and make them better.
SQL Optimization: This means finding the best way to ask the database a question. It’s like finding the shortest route on a map.
MySQL Performance: How well your MySQL database is working. A fast database means faster results.
Query Rewrite: Sometimes, the way you ask a question (a query) isn’t the best. Query rewriting is changing the question to get the same answer, but faster.
Index Optimization: An index is like the index in a book. It helps the database find information quickly. Index optimization means making sure your indexes are set up right.
Why is this important for AI Model Training?
AI models need lots and lots of data to learn. If your database is slow, it takes longer to get that data, which means:
For example, imagine you’re training a model to recognize cats in pictures. You have millions of pictures stored in your database. If it takes a long time to find those pictures, your cat-recognizing model will take a long time to learn!
Who is this guide for?
This guide is for Junior AI Model Trainers. You might be new to databases, but you need to understand how to make them work well with your AI projects.
Why 2025?
Databases are always changing! In the near future, we’ll be dealing with even more data than we do now. Also, new techniques are coming out to help databases run faster. This guide will help you use those new tools.
What will we cover?
We’ll learn about:
We won’t be talking about things like buying new computer hardware or setting up complicated database systems. We’re focusing on things you can do with the software you already have.
What to expect:
This guide is about doing. You’ll learn steps you can take right away to make your MySQL database faster for your AI model training.
What if I already know some SQL?
Great! Even if you know the basics, this guide will show you how to optimize your database specifically for AI workloads. Many beginners make mistakes like:
We’ll help you avoid these mistakes and become a MySQL performance pro!
Before you start making your MySQL database faster, you need to understand what kind of data you have and how you’re using it. This is like knowing what kind of ingredients you have before you start cooking!
Explain Data Profiling: Data profiling is like taking a close look at your data to understand it better. You find out things like what kind of data it is (numbers, words, dates), how many different values there are, and if there are any weird or missing pieces. Knowing this helps you choose the best way to organize and search your data, which makes everything faster. For example, if you know a column only contains a few different values, you might use a special type of index.
Tools for Data Profiling: You don’t have to do this by hand! MySQL has tools to help. You can use SQL queries (special questions you ask the database) to look at your data. MySQL Workbench, a free tool, also has features that can automatically profile your data.
Here’s an example of a simple SQL query to see how many different values are in a column called “model_type”:
|
|
This query tells you how many times each different “model_type” appears in your “ai_models” table.
Identifying Data Skew: Data skew means that some values in a column appear much more often than others. Imagine if 90% of your customers are in one city. If you search for customers in that city, it might take a long time because the database has to look through so much data.
You can find data skew with SQL queries too! For example:
|
|
This query shows you which “training_dataset” appears most often. If one dataset is used a lot more than others, you have data skew. You might also use graphs or charts to visualize this data.
Workload Analysis: Workload analysis means understanding how you’re using your database. What questions are you asking it? How often? Which questions take the longest? It’s like figuring out which recipes you cook most often so you can make sure you have the right ingredients and tools ready.
Tools for Workload Analysis: Tools like MySQL Enterprise Monitor or Percona Monitoring and Management (PMM) watch your database and keep track of all the queries that are running. They can show you which queries are slow or use a lot of resources. You look at the “query logs” - a record of all the questions asked to the database - to understand what’s happening.
Query Types and Frequencies: There are different kinds of questions you can ask a database:
Some of these questions are asked more often than others. For example, you might be selecting data to train your AI model much more often than you are inserting new data. Each type of query needs to be optimized in a different way.
Relating Data and Workload: Data profiling and workload analysis work together. Knowing what kind of data you have (data profiling) helps you understand why certain questions (workload analysis) are slow. For example, if you have data skew and you’re frequently asking for data from the most common value, that query will be slow.
Impact on AI Training: Let’s say you’re training an AI model to predict customer behavior. You need to select features (important pieces of information) from your customer database. This often involves running complex SQL queries with calculations (aggregate queries) to summarize the data. If you find that these aggregate queries are running slowly (workload analysis), and you also discover that some customer segments are much larger than others (data profiling), you can focus on optimizing those specific queries related to feature selection for the largest customer segments. This will have a big impact on how quickly you can prepare your data for AI training.
Indexes are like the index in a book. They help MySQL find the data it needs quickly, without having to read the entire table. Choosing the right indexes is super important for making your AI model training faster.
MySQL has different kinds of indexes. Each type is good for different kinds of searches. Let’s look at some common ones:
B-Tree Indexes: B-Tree indexes are the most common type. Think of them like a phone book, organized alphabetically. They are good for:
However, B-Tree indexes are not the best choice if you have data with many repeated values (low cardinality). For example, if you have a column that only has “yes” or “no” values, a B-Tree index might not help much.
Fulltext Indexes: Fulltext indexes are used for searching text. They are great for AI applications that involve:
For example, if you’re training an AI model to understand customer reviews, you can use a Fulltext index to quickly find reviews that mention specific products or features.
Spatial Indexes: Spatial indexes are used for data that represents locations on a map. They are useful for AI applications that involve:
For example, if you’re training an AI model to predict traffic patterns, you can use a Spatial index to quickly find data about roads and intersections in a specific area.
Hash Indexes: Hash indexes are very fast for finding exact matches, but they have limitations in MySQL. They are only available for the MEMORY
storage engine (which stores data in memory, not on disk). Because of this and other limitations, Hash indexes are generally not suitable for most AI workloads that require storing large amounts of data persistently.
Composite Indexes: Composite indexes are indexes on multiple columns. They are important for optimizing queries that filter data based on several criteria.
For example, let’s say you often search for data points based on their date and their label. You can create a composite index on the date
and label
columns. The order of the columns in the index matters! Put the column you filter on most often first.
|
|
This index will help MySQL quickly find data points that match specific dates and labels.
Covering Indexes: A covering index is an index that contains all the columns needed to answer a query. This means MySQL doesn’t have to go back to the main table to get the data, which makes the query much faster.
For example, let’s say you often run this query:
|
|
You can create a covering index on the date
, label
, and feature1
columns:
|
|
Now, when you run the query, MySQL can get all the data it needs directly from the index, without having to access the table.
Index Cardinality and Selectivity:
Indexes are most effective on columns with high cardinality and selectivity.
You can check the cardinality of an index using this SQL query:
|
|
The Cardinality
column in the output shows the estimated number of unique values in the index.
To calculate selectivity, you can divide the number of unique values in a column by the total number of rows in the table.
Index Maintenance:
Indexes can become fragmented over time, especially after you add, delete, or update a lot of data. This fragmentation can slow down your queries. It’s important to regularly maintain your indexes.
You can use the OPTIMIZE TABLE
command to rebuild an index and reduce fragmentation:
|
|
Important: OPTIMIZE TABLE
can take a long time, especially for large tables. Plan to run it during off-peak hours. Also consider using Percona Toolkit’s pt-online-schema-change
for online index rebuilds to minimize downtime.
Sometimes, the way you write your SQL query can make a big difference in how fast it runs. Query rewriting is like taking a sentence and making it shorter and easier to understand – but for computers!
Removing Redundant Joins: If you’re joining tables together, make sure you really need all those tables. Sometimes you can get the same information without joining as many tables. This makes the query faster.
Students
table, the Classes
table, and the Grades
table, but you only need the student’s name and grade, you might be able to skip joining the Classes
table.Simplifying WHERE
Clauses: The WHERE
clause tells MySQL what kind of data you want. If the WHERE
clause is too complicated, MySQL has to work harder. Try to make it simpler.
WHERE (age > 18 AND city = 'New York') OR (age > 21 AND city = 'Los Angeles')
, you could try breaking it into two separate queries and combining the results.UNION ALL
Instead of UNION
UNION
combines the results of two queries and removes any duplicates. UNION ALL
also combines the results, but it doesn’t remove duplicates. Removing duplicates takes extra time.UNION ALL
: If you know there won’t be any duplicates, or if you don’t care about duplicates, use UNION ALL
. It’s faster.UNION ALL
is a good choice.SELECT * FROM Orders WHERE customer_id IN (SELECT customer_id FROM Customers WHERE city = 'London')
, you could try SELECT Orders.* FROM Orders JOIN Customers ON Orders.customer_id = Customers.customer_id WHERE Customers.city = 'London'
.MySQL has lots of settings that you can change to make it run better. This is like tuning up a car engine.
innodb_buffer_pool_size
: This is the amount of memory MySQL uses to store data. The bigger it is, the faster MySQL can access data. Set it to a large value, but don’t use all of your computer’s memory!query_cache_type
and query_cache_size
: These settings control the query cache, which stores the results of queries so MySQL can quickly return them again if the same query is run. However, in newer versions of MySQL, the query cache has been deprecated, so consider alternatives like prepared statements or application-level caching.innodb_log_file_size
: This setting affects how quickly MySQL can write data to disk. A larger value can improve performance, but it also takes longer to recover from a crash.You’ve learned a lot about making your MySQL database faster for AI model training! Let’s recap the key things.
BTREE
or FULLTEXT
), create indexes that cover multiple columns (composite indexes), and keep your indexes up-to-date.EXPLAIN
), optimize subqueries, and use hints to tell MySQL how to run your query.WHERE
clauses, and use UNION ALL
instead of UNION
when you can.innodb_buffer_pool_size
to make it run better, and use connection pooling to reuse database connections.Making your database faster is something you always need to work on. Keep learning about new MySQL features and best practices. The MySQL documentation is a great place to start!
Try these techniques on your own AI model training workflows. Share what you learn with other people in the community. We can all learn from each other!
In the future, we might see computers using machine learning to automatically make queries faster. There might also be new kinds of indexes that are even better than the ones we have now.
Here are some helpful resources:
Good luck making your MySQL database super fast!
SQLFlash is your AI-powered SQL Optimization Partner.
Based on AI models, we accurately identify SQL performance bottlenecks and optimize query performance, freeing you from the cumbersome SQL tuning process so you can fully focus on developing and implementing business logic.
Join us and experience the power of SQLFlash today!.