SQL Server Performance Optimization: Window Functions to Double Your Query Efficiency!
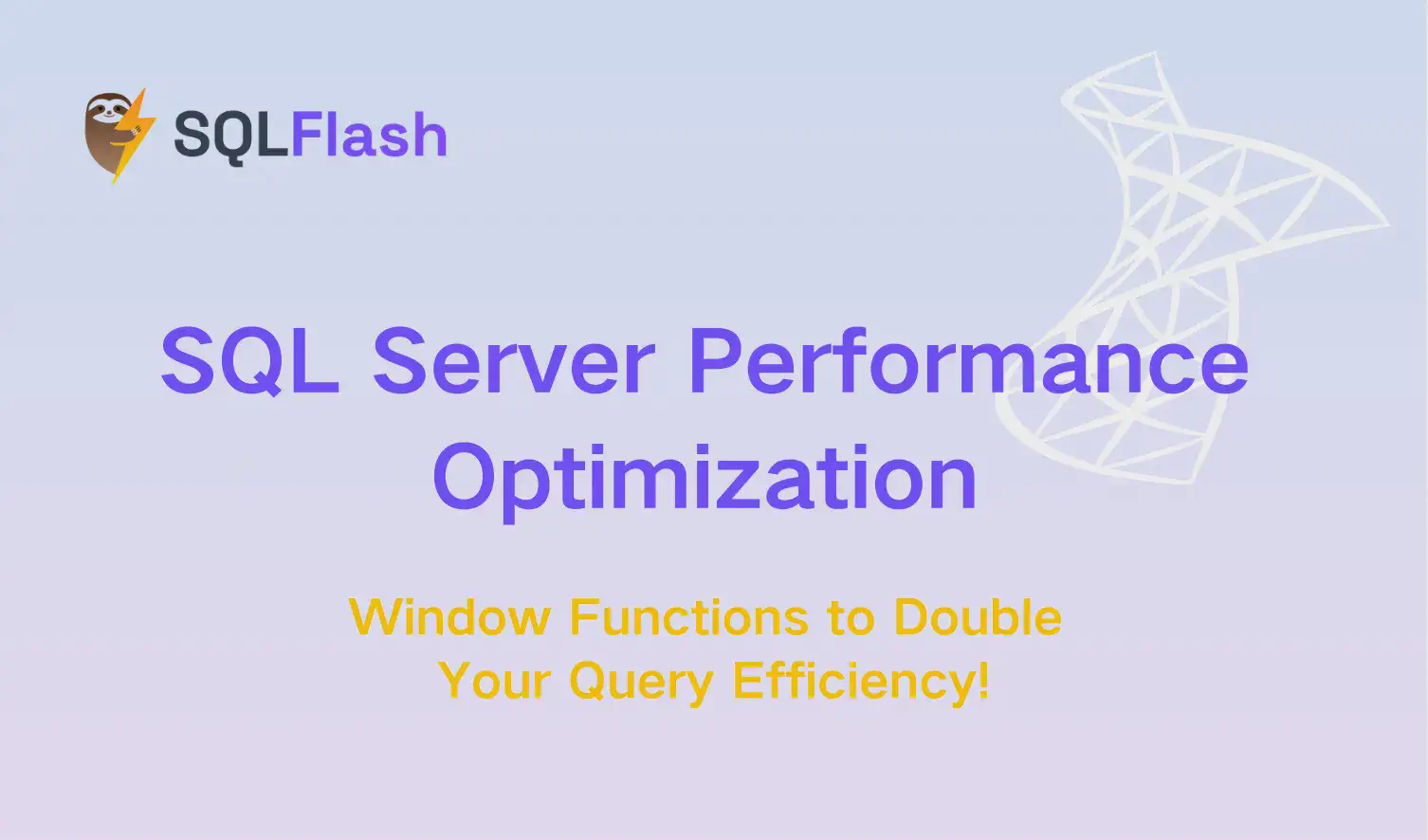
Hello everyone! Today, I want to share a practical SQL Server performance optimization technique: replacing subquery aggregations with window functions to boost query efficiency.
When writing SQL, we often need to add aggregated information to each row, such as calculating the total amount for each order or the average price. Many people (including my past self) start with subqueries:
|
|
This approach is straightforward, but it runs a subquery for every row, effectively scanning the table multiple times. While acceptable for small datasets, this method becomes inefficient with larger datasets.
Is there a more efficient way? Absolutely! Window functions allow you to calculate aggregated values for each row without using GROUP BY
. Here’s how the previous example can be rewritten using window functions:
|
|
This code is much cleaner, and performance improvements can be significant! Window functions execute in a single pass over the data, making them ideal for scenarios requiring row-level aggregations.
Assume we have an order_details
table, and we need to calculate the following metrics for each order (order_id
):
Developers often use correlated subqueries to achieve this:
|
|
This method executes two subqueries for each row, which can be inefficient, especially with large datasets.
Using window functions SUM()
, OVER()
, AVG()
, and OVER()
:
|
|
Window functions perform all calculations in a single table scan, avoiding redundant correlated subqueries. This results in significantly improved performance!
|
|
order_id
(total of 10,000 orders).total_amount
and avg_price
.order_id BETWEEN 1000 AND 2000
) and compare performance after filtering.SET STATISTICS IO, TIME ON
to capture logical reads, CPU time, and execution time.Metric | Subquery Aggregation | Window Function | Performance Improvement |
---|---|---|---|
Logical Reads (Pages) | 15,200 | 2,500 | 83% |
CPU Time (ms) | 4,300 | 1,200 | 72% |
Execution Time (ms) | 5,800 | 1,500 | 74% |
The data clearly shows that window functions outperform subquery aggregations by over 70%!
order_details
table, leading to a multiplicative increase in logical reads.PARTITION BY order_id
, all aggregate calculations are completed in a single scan.Official Documentation Recommendation:
“Window functions offer better performance for group aggregations, especially in large-scale data scenarios.”
Applicable Scenarios: SQL Server 2005 and above, where calculations need to attach aggregate values to each row.
Official Documentation Recommendation:
“Creating an index on the PARTITION BY
field can accelerate partitioning operations in window functions.”
Implementation:
|
|
Official Documentation Warning:
“Excessive partitioning fields can lead to decreased performance of window functions.”
Recommendation: Partition only by necessary fields (e.g., order_id
).
ORDER BY
SparinglyORDER BY
in window functions can lead to performance degradation (e.g., with ROWS BETWEEN
clauses). Use it only when necessary.In addition to manually rewriting SQL statements, tools like SQLFLASH can help automate optimization. SQLFLASH automatically analyzes SQL statements and replaces subqueries with window functions to enhance performance.
In simple terms, after using SQLFLASH, it automatically converts subquery-based SQL into a version that uses window functions, saving you the trouble of manual modification. The performance improvement is very significant.
By comparing the performance of subquery aggregations with window functions, we can draw the following conclusions:
It is recommended that developers prioritize window functions for implementing aggregation logic, provided version requirements are met, and further enhance performance with index optimization.
SQLFlash is your AI-powered SQL Optimization Partner.
Based on AI models, we accurately identify SQL performance bottlenecks and optimize query performance, freeing you from the cumbersome SQL tuning process so you can fully focus on developing and implementing business logic.
Join us and experience the power of SQLFlash today!.